Hello friends, in today’s post, we are starting you about HTML(Modal form validation), what happens. How does the key work, so today you should tell about HTML.
How do we do form validation in modal?
Hello friends, in today’s post we will tell you about form validation, how to apply form validation on modal from jQuery, for this you need some cdn so that this validation can be applied, then its cdn link is given below.
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <script type="text/javascript" src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> <script type="text/javascript" src="https://cdn.datatables.net/1.11.4/js/jquery.dataTables.min.js"> </script> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-validate/1.14.0/jquery.validate.min.js"> </script> <script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script>
After this, we have to use a bootstrap modal so that we can open the modal on button click, which is given below.
<button id="add-student-btn" data-toggle="modal" data-target="#modalForm"> Add Student </button> <!-- Modal --> <div class="modal fade" id="modalForm" role="dialog"> <div class="modal-dialog"> <div class="modal-content"> <form role="form" id='myForm'> <!-- Modal Header --> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal"> <span aria-hidden="true">×</span> <span class="sr-only">Close</span> </button> <h4 class="modal-title" id="myModalLabel">Add Student</h4> </div> <!-- Modal Body --> <div class="modal-body"> <p class="statusMsg"></p> <div class="form-group"> <label for="exampleInputPassword1" class="form-label">Email Id</label> <input type="text" class="form-control" id="add_name" name="add_name"> </div> <div class="form-group"> <label for="inputName">Name</label> <input type="text" class="form-control" id="inputName" name=" inputName" placeholder="Enter the Name here" /> </div> <div class="form-group"> <label for="inputEmail">Email</label> <input type="email" class="form-control" id="inputEmail" name="inputEmail" placeholder="Enter the email here" /> </div> <div class="form-group"> <label for="inputPhone">Phone</label> <input type="tel" class="form-control" id="inputPhone" name="inputPhone" placeholder="Enter the Phone Number here" /> </div> <div class="form-group"> <label for="inputstudent_id">Student ID</label> <input type="text" class="form-control" id="inputstudent_id" name="inputstudent_id" placeholder="Enter the Student ID here" /> </div> <div class="form-group"> <label for="status">Status:</label> <select id="status" name="status"> <option value="Active">Active</option> <option value="Inactive">Inactive</option> </select> </div> </div> <!-- Modal Footer --> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal">Close</button> <button type="submit" class="btn btn-primary submitBtn">Add Student</button> </div> </form> </div> </div> </div>
After this, the jQuery code is being given in which you can see that inputName is the name of the input box in the above modal so that when the input box is blank, the message of jQuery validation is shown.

The name of the input box below is defined in both the jQuery validation rules and the message below.

Now, Given below of jquery validation code
<script> $("#myForm").validate({ rules: { inputName: { required: true, letterswithspace: true }, add_name: { required: true }, inputEmail: { required: true, email: true }, inputPhone: { required: true, minlength: 10, maxlength: 10, digits: true } }, messages: { inputName: { required: "Please enter a name.", letterswithspace: "Please enter a valid name with letters and spaces only." }, add_name: { required: "Please enter a name." }, inputEmail: { required: "Please enter an email address.", email: "Please enter a valid email address." }, inputPhone: { required: "Please enter a phone number.", minlength: "Please enter a valid 10-digit phone number.", maxlength: "Please enter a valid 10-digit phone number.", digits: "Please enter a valid 10-digit phone number." } }, errorElement: "span", errorClass: "help-block", highlight: function(element, errorClass, validClass) { $(element).closest(".form-group").addClass("has-error"); }, unhighlight: function(element, errorClass, validClass) { $(element).closest(".form-group").removeClass("has-error"); } }); </script>
Now, you are being given its complete code below which you can see along with a form and its modal demonstration is given.
<html> <head> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <script type="text/javascript" src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> <script type="text/javascript" src="https://cdn.datatables.net/1.11.4/js/jquery.dataTables.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-validate/1.14.0/jquery.validate.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/sweetalert2@11"></script> </head> <body> <button id="add-student-btn" data-toggle="modal" data-target="#modalForm"> Add Student </button> <!-- Modal --> <div class="modal fade" id="modalForm" role="dialog"> <div class="modal-dialog"> <div class="modal-content"> <form role="form" id='myForm'> <!-- Modal Header --> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal"> <span aria-hidden="true">×</span> <span class="sr-only">Close</span> </button> <h4 class="modal-title" id="myModalLabel">Add Student</h4> </div> <!-- Modal Body --> <div class="modal-body"> <p class="statusMsg"></p> <div class="form-group"> <label for="exampleInputPassword1" class="form-label">Email Id</label> <input type="text" class="form-control" id="add_name" name="add_name"> </div> <div class="form-group"> <label for="inputName">Name</label> <input type="text" class="form-control" id="inputName" name="inputName" placeholder="Enter the Name here" /> </div> <div class="form-group"> <label for="inputEmail">Email</label> <input type="email" class="form-control" id="inputEmail" name="inputEmail" placeholder="Enter the email here" /> </div> <div class="form-group"> <label for="inputPhone">Phone</label> <input type="tel" class="form-control" id="inputPhone" name="inputPhone" placeholder="Enter the Phone Number here" /> </div> <div class="form-group"> <label for="inputstudent_id">Student ID</label> <input type="text" class="form-control" id="inputstudent_id" name="inputstudent_id" placeholder="Enter the Student ID here" /> </div> <div class="form-group"> <label for="status">Status:</label> <select id="status" name="status"> <option value="Active">Active</option> <option value="Inactive">Inactive</option> </select> </div> </div> <!-- Modal Footer --> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal">Close</button> <button type="submit" class="btn btn-primary submitBtn">Add Student</button> </div> </form> </div> </div> </div> <script> $("#myForm").validate({ rules: { inputName: { required: true, letterswithspace: true }, add_name: { required: true }, inputEmail: { required: true, email: true }, inputPhone: { required: true, minlength: 10, maxlength: 10, digits: true } }, messages: { inputName: { required: "Please enter a name.", letterswithspace: "Please enter a valid name with letters and spaces only." }, add_name: { required: "Please enter a name." }, inputEmail: { required: "Please enter an email address.", email: "Please enter a valid email address." }, inputPhone: { required: "Please enter a phone number.", minlength: "Please enter a valid 10-digit phone number.", maxlength: "Please enter a valid 10-digit phone number.", digits: "Please enter a valid 10-digit phone number." } }, errorElement: "span", errorClass: "help-block", highlight: function(element, errorClass, validClass) { $(element).closest(".form-group").addClass("has-error"); }, unhighlight: function(element, errorClass, validClass) { $(element).closest(".form-group").removeClass("has-error"); } }); </script> </body> </html>
this code output given below image
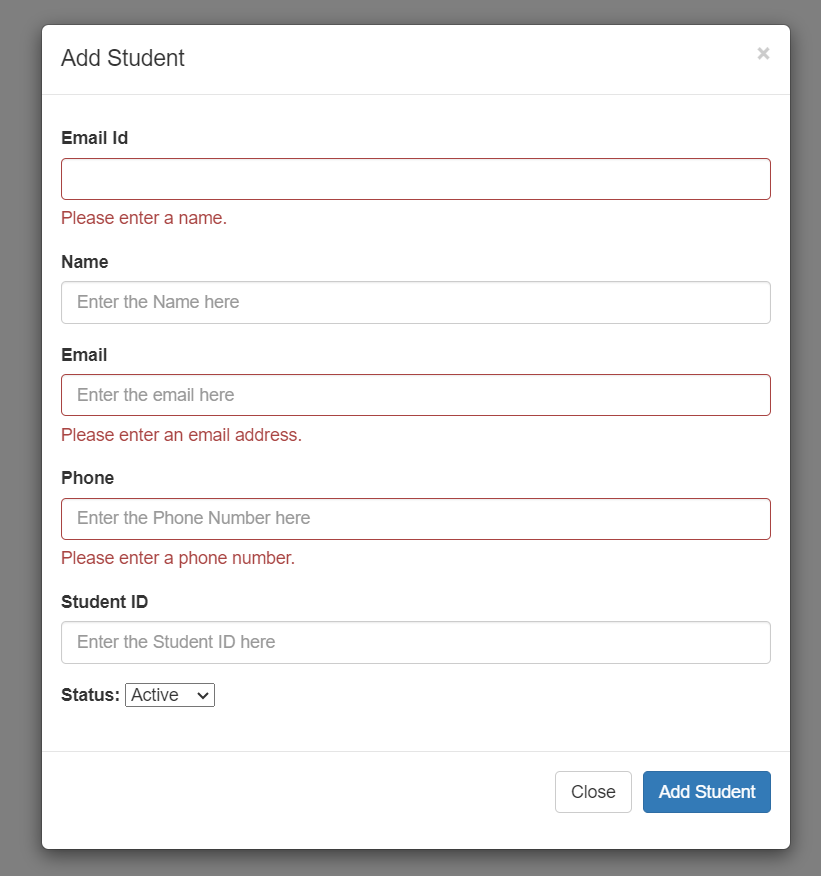
Request – If you found this post useful for you, then do not limit it to yourself, do share it.